How does a computer’s main memory differ from its auxiliary memory?
Main memory holds the current program and much of the data that the program is manipulating. The information stored in main memory typically is volatile, that is, it disappears when you shut down your computer.
Auxiliary memory, or secondary memory, exists even when the computer’s power is off.
After you use a text editor to write a program, will it be in main memory or auxiliary memory?
After you have closed the text editor it will be in auxiliary memory.
When a computer executes a program, will it be in main memory or auxiliary memory?
The program will be in main memory.
How does machine language differ from Java?
Machine language is the languange a computer can directly understand. Java is a programming language that is relatively easy for people understand and use. In order for a computer to run a Java program, it must first be translated into machine language.
How does bytecode differ from machine language?
When you compile a java class it is translated into bytecode. Bytecode is a machine language for a hypothetical computer know as a virtual machine. When you run a java program, the Java Virtual Machine (JVM) translates the bytecode into machine code.
What would the following statements, when used in a Java program, display on the screen?
int age;
age = 20;
System.out.println ("My age is");
System.out.println(age);
My age is
20
Write a statement or statements that can be used in a Java program to display the following on the screen:
3
2
1
System.out.println("3");
System.out.println("2");
System.out.println("1");
Write statements that can be used in a Java program to read your age, as entered at the keyboard, and display it on the screen.
java.util.Scanner keyboard = new java.util.Scanner(System.in);
System.out.println("Enter your age");
int age = input.nextInt();
System.out.println("Your age is " + age);
Given a person's year of birth, the Birthday Wizard can compute the year in which the person's nth birthday will occur or has occurred. Write statements that can be used in a Java program to perform this computation for the Birthday Wizard.
java.util.Scanner keyboard = new java.util.Scanner(System.in);
System.out.println("Enter the year you were born");
int yearBorn = keyboard.nextInt();
System.out.println("Enter birthday to check year for");
int birthday = keyboard.nextInt();
System.out.println("You will turn " + birthday + " in " + (yearBorn + birthday));
Write statements that can be used in a Java program to read two integers and display the number of integers that lie between them, including the integers themselves. For example, four integers are between 3 and 6: 3, 4, 5, and 6.
java.util.Scanner keyboard = new java.util.Scanner(System.in);
System.out.println("Enter the smaller integer");
int num1 = keyboard.nextInt();
System.out.println("Enter the larger integer");
int num2 = keyboard.nextInt();
for(int i = num1; i <= num2; i++)
{
System.out.println(i);
}
A single bit can represent two values: 0 and 1. Two bits can represent four values: 00, 01, 10, and 11. Three bits can represent eight values: 000, 001, 010, 011, 100, 101, 110, and 111. How many values can be represented by
(a)8 bits
(b)16 bits
(c)32 bits
(a)256
(b)65,536
(c)4,294,967,300
Find the documentation for the Java Class Library on the Oracle Web site. (At this writing, the link to this documentation is http://docs.oracle.com/javase/7/docs/api/.) Then find the description for the class Scanner. How many methods are described in the section entitled "Method Summary"?
55
Self-Test Question 27 asked you to think of some attributes for a song object. What attributes would you want for an object that represents a play list containing many songs?
Genre, Song Count, Total Time, List of Performers
What behaviors might a song have? What behaviors might a play list have? Contrast the difference in behavior between the two kinds of objects.
Song: Play, Pause, Stop
Playlist: Play All, Next Song, Previous Song, Remove Song
In the case of a song, behavior is for a single object (song). For a playlist, behavior is for a group of objects (songs).
What attributes and behaviors would an object representing a credit card account have?
Attributes: Card Type, Card Number, Card Holder's Name (First and Last), Card Holder's Address (Street, City, State and Zip), Expiration Date (Month and Year), Credit Limit, Balance
Behaviors: Make Payment, Refund Payment, Increase Credit Limit
Suppose that you have a number x that is greater than 1. Write an algorithm that computes the largest integer k such that 2^k is less than or equal to x.
x is greather than 1
k = 0
while 2^k is less than or equal to x
{
add 1 to k
}
subtract 1 from k
Write an algorithm that finds the maximum value in a list of values.
maxValue = -1
iterate through each list value
{
if list value is greater than maxValue then maxValue = list value
}
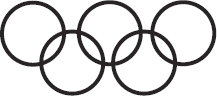
Write statements that can be used in a Java applet to draw the five interlocking rings that are the symbol of the Olympics. (Don't worry about the color.)
public void paint(Graphics g)
{
g.drawOval(40, 40, 120, 120);
g.drawOval(140, 40, 120, 120);
g.drawOval(240, 40, 120, 120);
g.drawOval(90, 130, 120, 120);
g.drawOval(190, 130, 120, 120);
}
Find the documentation for the class Graphics in the Java Class Library. (See Exercise 12.) Learn how to use the method drawRect. Then write statements that can be used in a Java applet to draw a square containing a circle. The circle's diameter and the square's side should be equal in size.
public void paint(Graphics g)
{
g.drawRect(10, 10, 100, 100);
g.drawOval(10, 10, 100, 100);
}
Write statements that can be used in a Java applet to draw the outline of a crescent moon.
public void paint(Graphics g)
{
g.drawArc(10, 10, 300, 300, 40 100);
g.drawArc(10, 20, 300, 240, 40, 100);
}